COMP 141 Fall 2019
Program 9: Memory
Assigned: Friday, November 22
Due: Monday, December 9, 2019 Tues, Dec. 10 by 11:55pm
You may work with a partner for this project. If you choose to do so, only turn in one copy of the project with both of your names in the comments at the beginning of the code.
If Memory doesn't particularly interest you, you may do one of the challenge problems at the end of this description, or propose a different project to me of comparable difficulty. You must get my approval before starting work on your project if you are not writing the Memory program.
The game Memory is a simple matching game usually played with cards in which there are 2 of each kind of card. The game starts with all the cards turned upside down (so you can't see their face), and a player will select two cards to turn over. If the two cards match, they remain up, otherwise, they are turned back over. The idea is to find all pairs of cards using one's memory of which cards they've seen before. Memory is normally a 2-player game such that the player with the most pairs at the end of the game, wins! We will instead implement a 1-player version, in which case the player will always "win" assuming they don't give up.
This program will most likely take longer than others this semester - be sure to START EARLY!
Program Specifications
This program requires you to use:
2-D lists
SimpleGraphics: open a canvas, use mouse clicks as the user input for moves, close the canvas
Random number generation: to initialize the board
Nested loops
Description
Write a program that
Uses simplegraphics to:
Prompt the user for the number of rows and columns on the board
Ensure that number of rows * number of columns is an even number (if not, reprompt the user)
Draw grid lines to display a grid with the correct number of rows and columns
Use clicks to determine which grid location a user has selected
Correctly uncover the appropriate "card" (i.e. display the correct shape/color/image for the clicked on grid square)
Allow the user to select 2 cards for each turn and if the cards match, leave them turned up, otherwise, turn them back over
You must correctly display the board after every click.
You will compare 2 cards and then on the next click, turn the cards back over if they are not matching.
You must use a variable sized board (you may assume you will never need more than 20 pairs.)
You must draw something in each grid when it is clicked, but it does not have to be a filled in circle.
Your program should keep running until there are no more unturned "cards".
Example Run 1
How many rows? 4
How many columns? 4
The rest of the program will be shown on the canvas,so here are some screenshots of a game while it is being played.
Initial Board |
Board After 1st turn |
End Board |
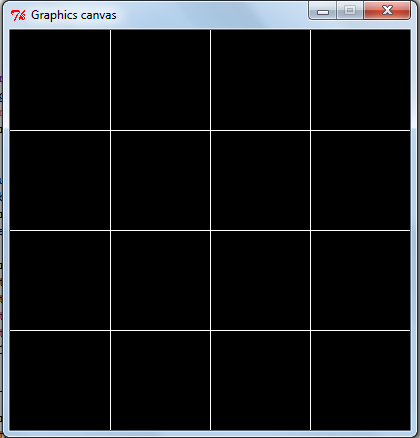 |
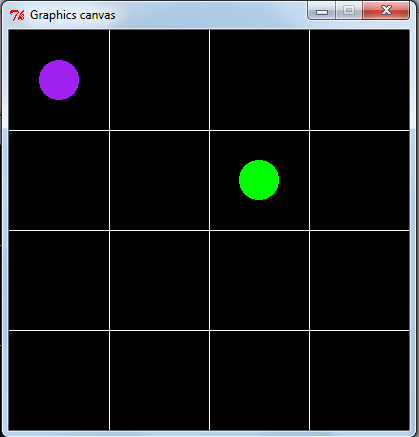 |
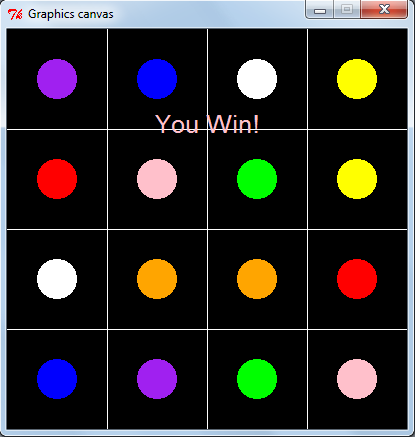 |
Example Run 2
How many rows? 2
How many columns? 6
The rest of the program will be shown on the canvas,so here are some screenshots of a game while it is being played.
Initial Board |
Board After 1st turn |
End Board |
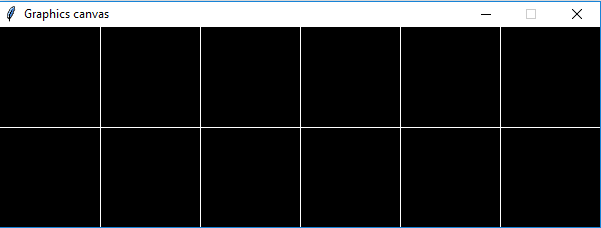 |
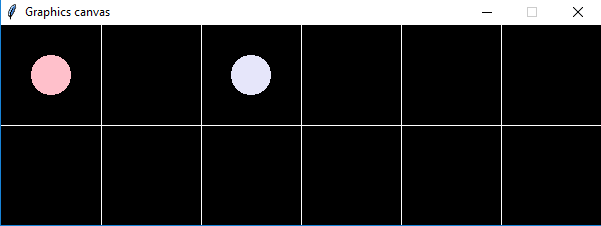 |
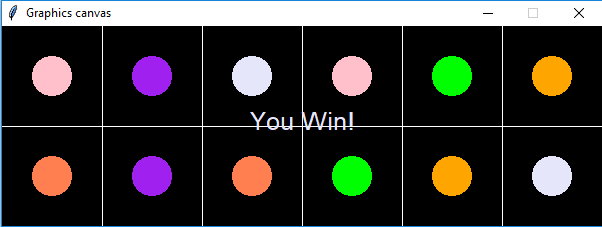 |
Your board does not need to look exactly like this, but all the mentioned functionality should work as shown.
Hints
Determine the number of pairs you'll need for your board (numPairs).
I suggest initializing your board with 2 copies each of 1-numPairs. (Don't randomly select the values, randomly select the row and column that value will go in.)
I suggest using positive values of 1 - numPairs to designate cards that are NOT showing, and negative values to designate cards that ARE showing.
I suggest making each grid space 100 x 100 pixels.
I suggest writing a do_move function that takes in the board, row and column and switches the sign of the value at that location in the board. (i.e. if it is -5 it becomes 5, if it is 5 it becomes -5.)
I suggest using a list to store color names; make this list have 20 values in it. Use the index into this list to associate a particular color with a number. For a complete list of all possible color names, see this.
I suggest dealing with a pair of clicks for each iteration of your loop, i.e., allow the user to click once and show that "card", click a 2nd time, show that "card", and then compare them and update the values in the board as necessary (switch back to positive if they were not matching.) This code is all in 1 iteration of the loop.
What to Do
Create a Python program named yourlastname_yourfirstname_prg9.py. If you work with a partner, name it yourlastname_partnerslastname_prg9.py
Include the standard program header at the top of your Python file.
Follow the comment guide to correctly comment your code.
Submit your Python file on Moodle under Program 9.
Note: If you include any images in your code, be sure to create a zip file with your .py file and the image files and submit the .zip file on Moodle.
Requirements
When I examine your program, an A program must satisfy the following requirements.
Your program file is named correctly and it includes the standard program header.
You must open the canvas.
You must draw grid lines on the canvas so the user can tell where each "card" is.
You must randomly initialize the board so that the game is different every time.
The user must be able to clearly tell when a pair is matching.
You must close the canvas on the last click.
You must turn over a card when the user clicks.
You must compare the 2 uncovered cards and turn them back over if they are not the same.
You must display a final message when the user "wins".
You must use appropriate functions (at least 3 functions besides main).
Creativity points - have fun with this and make it your own!
You must comment your code appropriately (particularly your functions), use good variable names, and your code must be neatly and clearly formatted and include proper use of 'white space'.
Alternate Challenge Questions
Here are some alternate games/projects with graphics that involve a 2-D list. You are welcome to work on one of these problems instead of Memory, but you MUST let me know as it is best to agree upon final functionality before you being implementing. As mentioned, you may also select a different game with graphics if none of these are to your liking either. Any ideas beyond this list MUST be approved before you begin working on them.
Minesweeper
Connect 4 (requires the normal board size of 6 x 7)
2048
Grading
Your program will be graded on correctness, as well as on coding style, which refers to choices you make when writing your code, such as good use of variable names, appropriate indentation,
and comments (this is not an exhaustive list). See the syllabus for further grading details.
You will receive one bonus point for every complete day your program is turned in early, up to a maximum of five points. For instance, if your program is due on September 20 at 11:59pm, if you turn in your code on Moodle any time on September 19 from 12:00am through 11:59pm, you will receive one extra point on your project. Programs submitted on September 18 from 12:00am through 11:59pm will receive two points. This pattern continues for up to five points.